How Do I Execute Terraform Commands in a Docker Container?
We may not want to install Terraform locally or on a VM. In such circumstances, making a container image containing Terraform binary is the best way to go.
💡
Tools used:
- Docker
- An AWS EC2 Ubuntu Instance
- Docker
- An AWS EC2 Ubuntu Instance
Step 1: Make a folder to hold the Terraform configuration file.
mkdir tf
cd tf
Step 2: Make a simple configuration file.
terraform {
required_version = "~> 1.0"
required_providers {
random = {
source = "hashicorp/random"
version = "3.5.1"
}
}
}
resource "random_string" "random" {
length = 16
}
output "random" {
value = random_string.random.length
}
The file above will:
- Set the required version for Terraform to be anything 1.0 and above.
- Set the required set of providers to hashicorp/random and version at 3.5.1.
- Declare a resource of type "random_string", name it "random" and set its length attribute to 16 characters.
- Finally, declare an output block and name it "random".
- The value assigned to this output is the length value.
Step 3: Make a Dockerfile at the root of the Terraform folder.
FROM golang:latest
ENV TERRAFORM_VERSION=1.2.5
RUN apt-get update && apt-get install unzip \
&& curl -Os https://releases.hashicorp.com/terraform/${TERRAFORM_VERSION}/terraform_${TERRAFORM_VERSION}_linux_amd64.zip \
&& curl -Os https://releases.hashicorp.com/terraform/${TERRAFORM_VERSION}/terraform_${TERRAFORM_VERSION}_SHA256SUMS \
&& curl https://keybase.io/hashicorp/pgp_keys.asc | gpg --import \
&& curl -Os https://releases.hashicorp.com/terraform/${TERRAFORM_VERSION}/terraform_${TERRAFORM_VERSION}_SHA256SUMS.sig \
&& gpg --verify terraform_${TERRAFORM_VERSION}_SHA256SUMS.sig terraform_${TERRAFORM_VERSION}_SHA256SUMS \
&& shasum -a 256 -c terraform_${TERRAFORM_VERSION}_SHA256SUMS 2>&1 | grep "${TERRAFORM_VERSION}_linux_amd64.zip:\sOK" \
&& unzip -o terraform_${TERRAFORM_VERSION}_linux_amd64.zip -d /usr/bin
RUN mkdir /tfcode
COPY . /tfcode
WORKDIR /tfcode
What's happening here?
The Dockerfile has instructions used for downloading Terraform executable files on a Linux machine.
Step 4: Build a Docker Image.
docker build -t terraform-container:v1.0
Step 5: Confirm the Image was created.
docker image ls

Step 6: Spin a Container from the terraform-container:v1.0 Image.
docker run -it -d --name my-tf-container terraform-container:v1.0 /bin/bash
Step 7: Confirm Container was created.
docker container ps -a

Step 8: Execute Terraform commands inside the my-tf-container Container.
docker exec my-tf-container terraform init
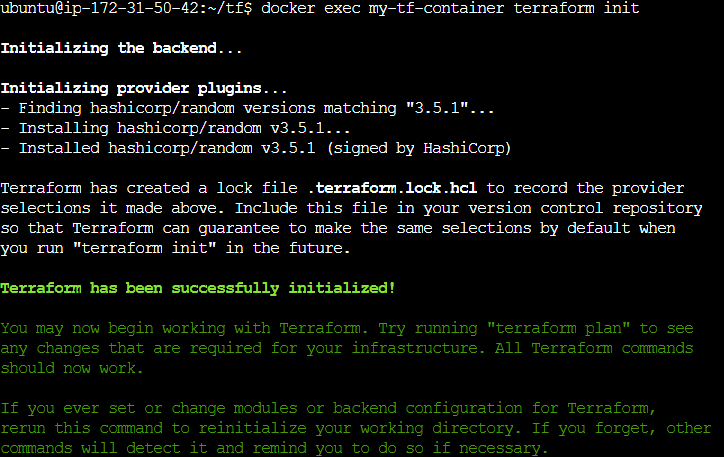
What's happening here?
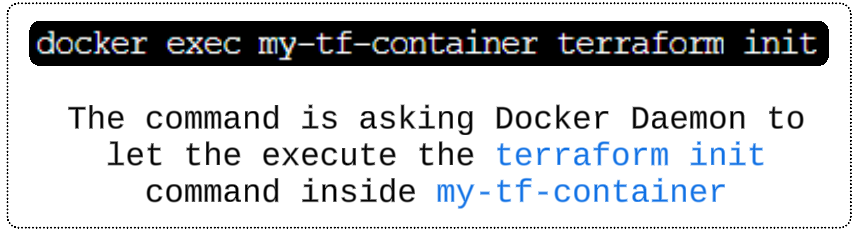
After this point, you may continue issuing other Terraform commands like plan, apply and destroy.
I write to remember, and if, in the process, I can help someone learn about Containers, Orchestration (Docker Compose, Kubernetes), GitOps, DevSecOps, VR/AR, Architecture, and Data Management, that is just icing on the cake.